Java FTP Download only structure of a directory from FTP server
- Details
- Written by Nam Ha Minh
- Last Updated on 19 July 2019   |   Print Email
import java.io.File; import java.io.IOException; import org.apache.commons.net.ftp.FTPClient; import org.apache.commons.net.ftp.FTPFile; /** * This utility class provides a method that downloads a structure * of a directory (excluding files) from a FTP server, using * Apache Commons Net API. * * @author www.codejava.net * */ public class FTPDownloadUtil { /** * Download structure of a directory from a FTP server. * @param ftpClient an instance of org.apache.commons.net.ftp.FTPClient class. * @param parentDir Path of the parent directory of the current directory being * downloaded. * @param currentDir Path of the current directory being downloaded. * @param saveDir path of directory where the whole remote directory will be * downloaded and saved. * @throws IOException if any network or IO error occurred. */ public static void downloadDirStructure(FTPClient ftpClient, String parentDir, String currentDir, String saveDir) throws IOException { String dirToList = parentDir; if (!currentDir.equals("")) { dirToList += "/" + currentDir; } FTPFile[] subFiles = ftpClient.listFiles(dirToList); if (subFiles != null && subFiles.length > 0) { for (FTPFile aFile : subFiles) { String currentFileName = aFile.getName(); if (currentFileName.equals(".") || currentFileName.equals("..")) { // skip parent directory and the directory itself continue; } if (aFile.isDirectory()) { String newDirPath = saveDir + parentDir + File.separator + currentDir + File.separator + currentFileName; if (currentDir.equals("")) { newDirPath = saveDir + parentDir + File.separator + currentFileName; } // create the directory in saveDir File newDir = new File(newDirPath); boolean created = newDir.mkdirs(); if (created) { System.out.println("CREATED the directory: " + newDirPath); } else { System.out.println("COULD NOT create the directory: " + newDirPath); } // download the sub directory downloadDirStructure(ftpClient, dirToList, currentFileName, saveDir); } } } } }And here is code of a test program:
import java.io.IOException; import org.apache.commons.net.ftp.FTPClient; public class DownloadDirectoryStructureTest { public static void main(String[] args) { String server = "www.codejava.net"; int port = 21; String user = "username"; String pass = "password"; FTPClient ftpClient = new FTPClient(); try { // connect and login to the server ftpClient.connect(server, port); ftpClient.login(user, pass); // use local passive mode to pass firewall ftpClient.enterLocalPassiveMode(); System.out.println("Connected"); String remoteDirPath = "/Test"; String saveDirPath = "E:\\Download"; FTPDownloadUtil.downloadDirStructure(ftpClient, remoteDirPath, "", saveDirPath); // log out and disconnect from the server ftpClient.logout(); ftpClient.disconnect(); System.out.println("Disconnected"); } catch (IOException ex) { ex.printStackTrace(); } } }Compile the utility class and the test program:
javac -cp commons-net-VERSION.jar;. DownloadDirectoryStructureTest.java
Run the test program:java -cp commons-net-VERSION.jar;. DownloadDirectoryStructureTest
Suppose the directory /Test on the FTP server has the following structure: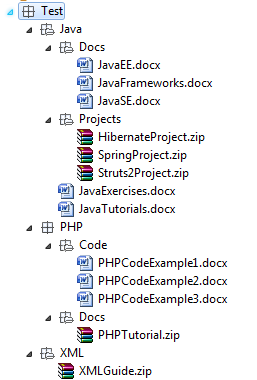
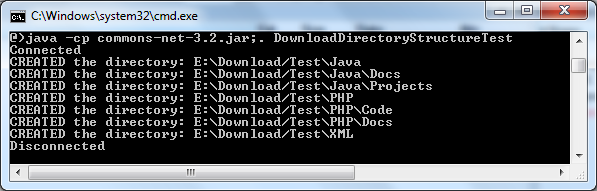
Related Java FTP Tutorials:
- How to download a complete folder from a FTP server
- Upload only structure of a directory to FTP server
- Java FTP file download tutorial and example
- Use URLConnection to download file from FTP server
Other Java FTP Tutorials:
- Connect and login to a FTP server
- Java FTP create directory example
- Java FTP example - Change working directory
- Java FTP list files and directories example
- Java FTP file upload tutorial and example
- Java FTP delete file example
- Java FTP example - Search for files and directories
About the Author:

Comments