- Details
- Written by Nam Ha Minh
- Last Updated on 06 July 2019   |   Print Email
JPasswordFieldis a subclass of the
JTextField so it acts like an ordinary text field but it hides the actual characters typed by showing a series of echo characters such as asterisks (*) or a dots, for security purpose. For example:

In this article, we provide a summary of the common and recommended practices when working with
JPasswordField in Swing, with code examples and a demo program.
Table of content:
- Creating new JPasswordField object
- Adding the password field to a container
- Setting and getting password
- Adding event listeners
- Selecting text
- Customizing appearance
- A demo program for JPasswordField
A typical usage of
JPasswordField looks like this:
// create new object
JPasswordField passwordField = new JPasswordField(20);
// add to the container
frame.add(passwordField);
// get the password
char[] password = passwordField.getPassword();
Let’s dive into more details.
1. Creating new JPasswordField object
When creating a new
JPasswordField object, we can specify an initial text and the field’s width in number of columns, using one of the following constructors:
JPasswordField(int columns)
JPasswordField(String text)
JPasswordField(String text, int columns)
Note that the parameter
columns specifies minimum width of the field in a number of columns, not in number of pixels nor characters. Here are some examples:
JPasswordField passwordField = new JPasswordField(20);
JPasswordField passwordField = new JPasswordField("secret");
JPasswordField passwordField = new JPasswordField("secret", 20);
We can also use an empty constructor to create new object and then set the columns and initial password later, for example:
JPasswordField passwordField = new JPasswordField(20);
passwordField.setColumns(10);
passwordField.setText("secret");
NOTE: Like other Swing components, the
JPasswordField class resides in the package
javax.swing.
2. Adding JPasswordField to a container:
The password field cannot stand alone. It must be added to a parent container such as
JFrame or
JPanel. For example:
frame.add(passwordField);
panel.add(passwordField);
Adding to a
JFrame with
BorderLayout:frame.add(passwordField, BorderLayout.NORTH);
Adding to a
JFrame with
GridBagLayout:GridBagConstraints constraints = new GridBagConstraints();
// set constraints...
frame.add(passwordField, constraints);
3. Setting and getting password from JPasswordField
Use the
setText() method (inherited from
javax.swing.text.JTextComponent class) to set password for the field:
passwordField.setText("secret");
To retrieve password typed into the field, use the
getPassword() method:
char[] password = passwordField.getPassword();
For security purpose, the
getPassword() method returns a
char array instead of a
String object. So it’s
RECOMMENDED to compare the password as follows:
char[] password = passwordField.getPassword();
char[] correctPass = new char[] {'s', 'e', 'c', 'r', 'e', 't'};
if (Arrays.equals(password, correctPass)) {
System.out.println("Password is correct");
} else {
System.out.println("Incorrect password");
}
In the above code snippet, we use the method
equals() of the
java.util.Arrays class to compare two
char arrays.
AVOID getting the password as follows:
String password = new String(passwordField.getPassword());
AVOID comparing the password as follows:
String password = new String(passwordField.getPassword());
if (password.equals("secret")) {
// password is correct
}
It’s also recommended to clear the
char array when we are finished using it (for enhanced security):
Arrays.fill(correctPass, '0');
NOTE: The
getText() method is deprecated in
JPasswordField class because it returns password as a
String object which might be vulnerable for security.
4. Adding event listeners for JPasswordField
We can add a listener for the event in which the user presses
Enter key after typing text into the field. For example:
passwordField.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent event) {
JPasswordField field = (JPasswordField) event.getSource();
char[] password = field.getPassword();
if (password.length < 8) {
System.out.println("Password must contain at least 8 characters!");
}
}
});
The above code will check for length of the entered password as soon as the user hits
Enter key.We can also add a listener to handle key events which are fired whenever the user types a character into the field. For example:
passwordField.addKeyListener(new KeyListener() {
@Override
public void keyTyped(KeyEvent event) {
// do something when a key has been typed
}
@Override
public void keyReleased(KeyEvent event) {
// do something when a key has been released
}
@Override
public void keyPressed(KeyEvent event) {
// do something when a key has been pressed
}
});
A typical usage of key event listener is to check emptiness of the field in order to enable/disable the action button accordingly, for example:
passwordField.addKeyListener(new KeyAdapter() {
@Override
public void keyReleased(KeyEvent event) {
JPasswordField field = (JPasswordField) event.getSource();
char[] password = field.getPassword();
if (password == null || password.length == 0) {
actionButton.setEnabled(false);
} else {
actionButton.setEnabled(true);
}
}
});
Notice in the above code snippet, we create an anonymous class that extends the
KeyAdapter class, instead of implementing the
KeyListener interface, so we can override only the interested method.
5. Selecting text in JPasswordField
Select all text in the field:
passwordField.selectAll();
The selection looks like this:

6. Customizing appearance of JPasswordField
- Set echo character:
passwordField.setEchoChar('*');
Image:

- Set font style, background and foreground colors:
passwordField.setFont(new java.awt.Font("Arial", Font.BOLD, 20));
passwordField.setBackground(Color.YELLOW);
passwordField.setForeground(Color.BLUE);
Image:

- Set tooltip text:
passwordField.setToolTipText("Password must contain at least 8 characters");
Image:
7. A demo program for JPasswordField
For reference, we created a sample program looks like this:
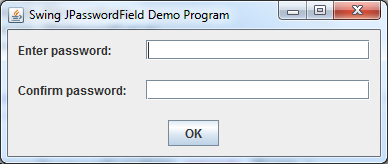
This program displays two password fields and verifies if the passwords are matched and correct.On clicking the
OK button, if the two passwords are not matched, the following message appears:
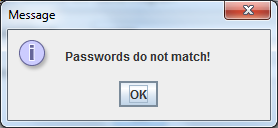
If the two passwords are matched, but do not equal to “codejava”, this message appears:
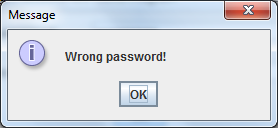
And if the both passwords equal to “codejava”, this message appears:
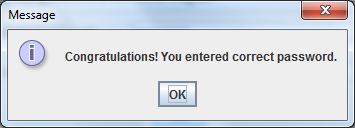
You can download full source code of the demo program as well as its runnable jar file in the attachments section below.
Other Java Swing Tutorials:
About the Author:
Nam Ha Minh is certified Java programmer (SCJP and SCWCD). He started programming with Java in the time of Java 1.4 and has been falling in love with Java since then. Make friend with him on
Facebook and watch
his Java videos you YouTube.