Java Shell (jshell) Tutorial
- Details
- Written by Nam Ha Minh
- Last Updated on 04 August 2019   |   Print Email
1. Start jshell
The Java Shell comes with JDK 9 so you need to have JDK 9 installed on your computer. If the PATH environment variable is configured properly, you can type jshell anywhere in the command line prompt:jshell
Otherwise you can go to JDK’s installation directory, go in the bin folder and open the jshell program, for example (on Windows):c:\Program Files (x86)\Java\jdk-9\bin>jshell
Then you see a welcome message and the command prompt changes to jshell>: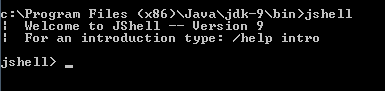
/help intro
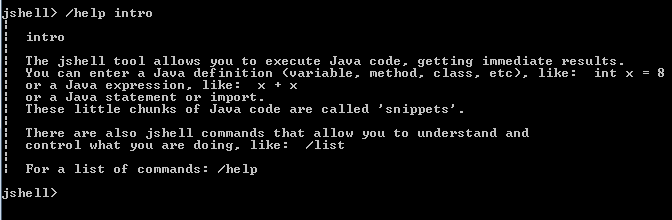
These little chunks of Java code are called 'snippets'.
That means what you type is called snippets and these snippets are given identifiers (ID) so you can edit or drop them later on.Now you’re in the Java Shell. Read the next sections to see how to use it.2. Execute Java Expressions and Statements
Basically, you can type any valid expressions and statements in the Java language, the shell gives you results immediately, for example:jshell> 123 + 456 $1 ==> 579This evaluates the expression ‘123 + 456’ and gives the result: 579. The result is stored in a variable named $1.Similarly, you can concatenate two String literals like this:
jshell> "Java " + "World" $2 ==> "Java World"And the result is assigned to a new variable named $2, implicitly.You can test the substring() method on a String literal like this:
jshell> "Java World".substring(0, 4) $3 ==> "Java"You see, the result String is stored in a variable named $3, implicitly. You can declare a variable just like normal Java code:
jshell> List<String> list = new ArrayList<>() list ==> []This creates a new ArrayList collection, and the feedback tells us that the list is initially empty.The shell can remember all variables you declared, so you can add elements to the above list like this:
jshell> list.add("Apple") $5 ==> true jshell> list.add("Banana") $6 ==> true jshell> list.add("Something") $7 ==> trueAnd simply type the variable name to see its value:
jshell> list list ==> [Apple, Banana, Something]Here, the elements in the list collection are printed out. Now you can investigate other methods of the List interface (the declared type of the variable list), for example:
jshell> list.size() $9 ==> 3 jshell> list.get(2) $10 ==> "Something" jshell> list.get(5) | java.lang.IndexOutOfBoundsException thrown: Index 5 out-of-bounds for length 3 | at Preconditions.outOfBounds (Preconditions.java:64) | at Preconditions.outOfBoundsCheckIndex (Preconditions.java:70) | at Preconditions.checkIndex (Preconditions.java:248) | at Objects.checkIndex (Objects.java:372) | at ArrayList.get (ArrayList.java:439) | at (#11:1) jshell> list.remove(2) $12 ==> "Something" jshell> list list ==> [Apple, Banana]You can see the statement list.get(5) throws an exception because the list contains only 3 elements. Very useful and rapid to learn Java programming with jshell, right?At anytime, you can type /varsto list all the declared variables and their values. For example:
jshell> /vars | int $1 = 579 | String $2 = "Java World" | String $3 = "Java" | List<String> list = [Apple, Banana] | boolean $5 = true | boolean $6 = true | boolean $7 = true | int $9 = 3 | String $10 = "Something" | String $11 = null | String $12 = "Something"
3. Declare a Java Method
The Java Shell allows to declare a method without writing any enclosing class and invoke the method later. For example:jshell> public double triangleSquare(int base, int height) { ...> return 0.5 * base * height; ...> } | created method triangleSquare(int,int)Press Enter after each line and the shell can automatically detect when a method completes.This declares a method that calculates the area of a triangle given its base and height. Then you can invoke the method directly without creating a new instance of a class:
jshell> triangleSquare(5, 8) $15 ==> 20.0You see? This is very convenient to test a method rapidly.And you can type/methods command to see all the declared methods. For example:
jshell> /methods | double triangleSquare(int,int)If you want to redefine the method, simply type the method declaration again (use the Up/Down arrow keys to reuse previous inputs). You will see the feedback ‘modified method…’ - something like this:
| modified method triangleSquare(int,int)
4. Declare a Java Class
Similarly to method declaration, you can declare a class by typing its code just like you write Java code in an editor, and the shell can detect when the class completes. For example:jshell> class Person { ...> String name; ...> int age; ...> ...> public void say() { ...> System.out.println("Hello, my name is: " + name); ...> } ...> } | created class PersonThen you can test the class like this:
jshell> Person p = new Person() p ==> Person@10587f1 jshell> p.name = "John" $18 ==> "John" jshell> p.age = 33 $19 ==> 33 jshell> p.say() Hello, my name is: JohnTo see all the declared classes, type /types command in the shell. For example:
jshell> /types | class PersonIf you want to redefine the class, simply type the class declaration again (use the Up/Down arrow keys to reuse previous inputs). You will see the feedback ‘modified class…’ - something like this:
| modified class Person
5. Declare a Java Interface
Similar to class declaration, you can declare an interface and the shell can detect when the declaration completes. Here’s an example:jshell> public interface Animal { ...> public void move(); ...> public void eat(); ...> public void sleep(); ...> } | created interface AnimalHere, we declare the interface Animal with three methods. Now try to create a class that implements this interface like this:
jshell> class Dog implements Animal { ...> } | Error: | Dog is not abstract and does not override abstract method sleep() in Animal | class Dog implements Animal { | ^----------------------------...We got an error saying that the Dog class doesn’t override the abstract methods defined by the Animal interface. Very useful for learning the Java language, right?The declared interfaces also listed when typing the /types command:
jshell> /types | class Person | interface AnimalYou can redefine the interface by retyping its declaration (using Up/Down arrow keys to reuse previous inputs). You can see the feedback ‘replaced interface…’ - something like this:
| replaced interface Animal
6. Using Java Imports
By default, jshell imports some common packages on startup. You can type /importscommand to see all the available imports:jshell> /imports | import java.io.* | import java.math.* | import java.net.* | import java.nio.file.* | import java.util.* | import java.util.concurrent.* | import java.util.function.* | import java.util.prefs.* | import java.util.regex.* | import java.util.stream.*Note that though the java.lang package is not listed here, it is also imported by default.The following example shows two packages java.util.nio and java.sql:
jshell> import java.nio.*; jshell> import java.sql.*;or import a specific type:
jshell> import java.sql.ConnectionType /imports command again and you will see the recent import statements are listed:
jshell> /imports | import java.io.* | import java.math.* | import java.net.* | import java.nio.file.* | import java.util.* | import java.util.concurrent.* | import java.util.function.* | import java.util.prefs.* | import java.util.regex.* | import java.util.stream.* | import java.nio.* | import java.sql.* | import java.sql.Connection
7. View History
You can view the history of what you typed since the shell was launched, using the /history command. For example:jshell> /history 123 + 456 "Java " + "World" "Java World".substring(0, 4) List<String> list = new ArrayList<>() list.add("Apple") list.add("Banana") list.add("Something") list.siz() list.size() list.get(5) list.remove(2) list /vars public double triangleSquare(int base, int height) { return 0.5 * base * height; } triangleSquare(5, 8) /methods /importsThe history is stored in the current session only. If you quit the shell, the history is cleared.
8. Use Tab Completion
One interesting and helpful feature of jshell is code auto completion and API documentation. When entering code in the shell, you can use the Tab key to automatically complete an item, or show a list of possible options.For example, if there’s a method named triangleSquare(), you can just type the triangle and press the Tab key, the shell automatically completes the method name:jshell> /methods | double triangleSquare(int,int) jshell> triangle (Press Tab key)Then the shell automatically completes the method name:
jshell> triangleSquare( triangleSquare( jshell> triangleSquare(You can also use the Tab key to show different signatures of methods or constructors, along with their documentation.For example, enter the following declaration:
jshell> List<String> items = new ArrayList<>(When typed the open parenthesis, press the Tab key and the shell shows a list of signatures of constructors of the ArrayList class:
jshell> List<String> items = new ArrayList<>( $8 list names Signatures: ArrayList<E>(int initialCapacity) ArrayList<E>() ArrayList<E>(Collection<? extends E> c) <press tab again to see documentation> jshell> List<String> items = new ArrayList<>(Now, press the Tab key you will see the documentation of the first signature:
jshell> List<String> items = new ArrayList<>( ArrayList<E>(int initialCapacity) Constructs an empty list with the specified initial capacity. Parameters: initialCapacity - the initial capacity of the list Thrown Exceptions: IllegalArgumentException - if the specified initial capacity is negative <press tab to see next documentation> jshell> List<String> items = new ArrayList<>(It’s very useful, isn’t it? Press the Tab key again you will the documentation of the next signature, and so on.
9. List the code snippets you have typed
If you want to review all the code snippets you have typed, type /list command. For example:jshell> /list 1 : public double triangleSquare(int base, int height) { return 0.5 * base * height; } 2 : triangleSquare(5, 8) 3 : class Person { String name; int age; public void say() { System.out.println("Hello, my name is: " + name); } } 4 : Person p = new Person(); 5 : p.name = "John" 6 : p.age = 33 7 : p.say() 8 : List<String> list = new ArrayList<>(); 9 : list.add("Apple") 10 : list.add("Banana") 11 : list.add("Something")Note that there are numbers on the right act as snippet IDs, so you can use these IDs for further operations. For example, the following command shows only source code of the Person class (the code snippet of this class has ID 3 as shown above):
jshell> /list 3 3 : class Person { String name; int age; public void say() { System.out.println("Hello, my name is: " + name); } }The /list <id> command allows you to list the snippet with the specified snippet ID.You can also use the /list <name> command to list the code of the given item name. For example, the following command shows the code of the Person class:
/list Person
10. Drop a Snippet
You can drop a snippet you have typed by using the command /drop <name> or /drop <id>. <name> can be variable name, method name or class name; and <id> is the ID of the snippet you see using the /list command.For example, the following command removes the declaration of the Person class:jshell> /drop Person | dropped class PersonThat means you can no longer use the Person class and any reference variables of this class.Suppose the /list command shows the following snippets:
jshell> /list 2 : triangleSquare(5, 8) 4 : Person p = new Person(); 5 : p.name = "John" 6 : p.age = 33 7 : p.say() 8 : List<String> list = new ArrayList<>(); 9 : list.add("Apple") 10 : list.add("Banana") 11 : list.add("Something")Then you can use the command /drop 8 or /drop listto remove the declaration of the variable list:
jshell> /drop 8 | dropped variable list
11. Edit a code snippet in an external editor
You can use the command /edit <id> to edit a code snippet with the specified snippet ID in an external editor. For example, to edit the snippet of the Person class (snippet ID = 3):jshell> /edit 3Then jshell opens an external editor looks like this:
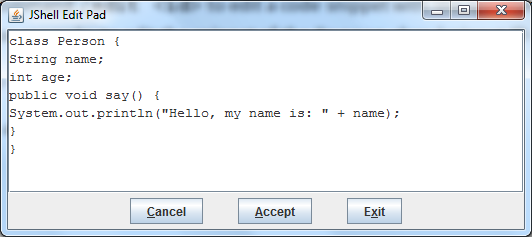
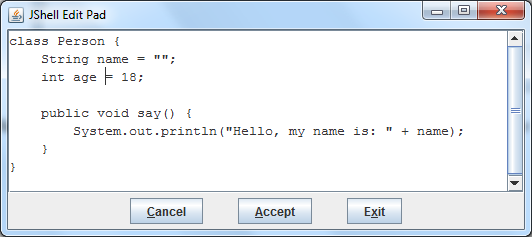
jshell> /edit 3 | modified class PersonYou can use the /list command to see the updated code:
jshell> /list 1 : public double triangleSquare(int base, int height) { return 0.5 * base * height; } 2 : triangleSquare(5, 8) 4 : Person p = new Person(); 5 : p.name = "John" 6 : p.age = 33 7 : p.say() 8 : List<String> list = new ArrayList<>(); 9 : list.add("Apple") 10 : list.add("Banana") 11 : list.add("Something") 12 : class Person { String name = ""; int age = 18; public void say() { System.out.println("Hello, my name is: " + name); } }But now, the snippet ID of the updated code has changed.Note that you can also use the /edit <name> command to edit a code snippet. For example, the following command edits code of the Person class:
/edit Person
12. Open a Source File
The Java Shell provides the /open <filename> command that allows you to open a file and read its contents and snippets and command, which is very useful for reusing commands or code. The file can contains any Java code and valid jshell’s commands.For example, the following command imports the code in Person.java file to the shell:jshell> /open E:\Java\Person.javaYou can also use the /open command with some predefined scripts:/open DEFAULT: loads the default imports./open JAVASE: imports all JavaSE packages./open PRINTING: uses print, println and printf methods as jshell methods.
13. Save Snippets to a File
You can save the snippets or commands you have typed to a specified file, using the following command:/save <file>For example, the following command saves snippets and commands to a file named snippets.txt in E:\Java directory:
/save E:\Java\snippets.txtType the command /help save to see additional options for the /save command.
14. Using Help
Type /help to list all the commands supported by jshell.And type /help <command>to see the usage of a specific command, for example:jshell> /help /history | | /history | | Display the history of snippet and command input since this jshell was launched.You can also use the ? as shortcut for help, for example:
/? /history
15. Use Startup Options
You can specify options when launching jshell, for examples:- loading scripts from files- specify directories and JAR files in classpath- send flags to the compiler and runtime system- set feedback mode (normal, verbose or concise)...etcSee the options details in jshell Tool Reference.16. Exit jshell
Type /exit to end the session and quit the shell:jshell> /exit | Goodbye c:\Program Files (x86)\Java\jdk-9\bin>For more information about using the Java Shell, refer to the following documents:
References:
Other Java Tools Tutorials:- javac command examples
- java command examples
- Java jar command examples
- Java serialver command examples
- Understanding the triad tools javac, java and jar in JDK
- How to compile, package and run a Java program using command-line tools (javac, jar and java)
About the Author:

Comments