How to use Cookies in Java web application
- Details
- Written by Nam Ha Minh
- Last Updated on 28 June 2019   |   Print Email
2. How to read cookies
3. How to update a cookie
4. How to delete a cookie
5. Java Cookies Example Project
1. How to create a cookie
To store a cookie in the web browser, first create a new Cookie object:String name = "Cookie name"; String value = "Cookie value"; Cookie cookie = new Cookie(name, value);Then call the addCookie() method of the HttpServletResponseobject in a Servlet class like this:
response.addCookie(cookie);This will send appropriate HTTP headers to the client, and the browser stores the cookie on user’s computer.
cookie.setComment("This cookie stores username of the customer");setDomain(String): specifies the domain name within which this cookie is visible. By default, cookies are only returned to the server that sent them. So setting the domain name makes the cookies available for different servers under the same domain name. For example:
cookie.setDomain(".codejava.net");This cookie will be available for all servers under the domain codejava.net. Note that the domain name should starts by a dot. The following example sets domain of the cookie to localhost:
cookie.setDomain("localhost");setHttpOnly(boolean): if set to true, Javascript can’t read this cookie on the client side, which can prevent some kinds of cross-site scripting attacks. For example:
cookie.setHttpOnly(true);setMaxAge(int): specifies how long the cookie is stored in user’s computer, in seconds. If not set, the cookie is deleted when the web browser exits. For example:
cookie.setMaxAge(7 * 24 * 60 * 60);This sets the cookie’s life is 7 days (= 24 hours x 60 minutes x 60 seconds) and it is still stored on the user’s computer when the browser exists. setPath(String): use this method if you want to restrict the cookie to be available for a certain path (and its subpaths) on the server. For example:
cookie.setPath("/product");setSecure(boolean): if set to true, the cookie is sent from the browser to the server only when using secure protocol (HTTPS or SSL). Default is false.
2. How to read cookies
To read cookies sent from the browser to the server, call getCookies() method on a HttpServletRequest object in a Java servlet class. This method returns an array of Cookie objects that are visible to the current request. For example, the following code read all cookies and print its names and values:Cookie[] cookies = request.getCookies(); PrintWriter writer = response.getWriter(); for (Cookie aCookie : cookies) { String name = aCookie.getName(); String value = aCookie.getValue(); writer.println(name + " = " + value); }That means if you want to read a specific cookie, you need to check the cookie’s name in the loop. For example:
String username = null; for (Cookie aCookie : cookies) { String name = aCookie.getName(); if (name.equals("username")) { username = aCookie.getValue(); break; } }As you can see, this code snippet reads the value of the cookie having the name “username”.
3. How to update a cookie
To update an existing cookie, you need to create a new cookie with the same name and add it to the response. For example:String name = "Cookie name"; String value = "New value"; Cookie cookie = new Cookie(name, value); response.addCookie(cookie);This will overwrite (update) the cookie with the same name.
4. How to delete a cookie
To remove a cookie from the browser’s cache, you need to create a new cookie with the same name, set its max age to zero and add it to the response. For example:Cookie cookie = new Cookie("username", ""); cookie.setMaxAge(0); response.addCookie(cookie);This code deletes the cookie with name “username” from the browser.
5. Java Cookies Example Project
The following sample project helps you learn how to use cookies in a Java web application. Create a simple Java web project. Code the home page (index.jsp) as follows:<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%> <!DOCTYPE html> <html> <head> <meta charset="ISO-8859-1"> <title>Java Cookies Example</title> </head> <body> <div align="center"> <h1>Java Cookies Example</h1> <h2><a href="/add_cookies">Add Cookies</a></h2> <h2><a href="/read_cookies">Read Cookies</a></h2> <h2><a href="/delete_cookies">Delete Cookies</a></h2> </div> </body> </html>This page displays 3 hyperlinks to test create, read and delete cookies:
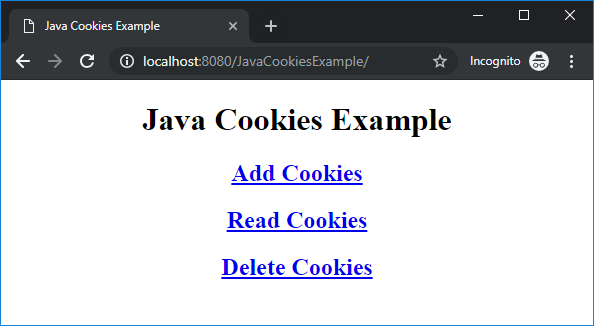
package net.codejava; import java.io.IOException; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.Cookie; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; @WebServlet("/add_cookies") public class AddCookiesServlet extends HttpServlet { private static final long serialVersionUID = 1L; private static int cookieCount; public AddCookiesServlet() { } protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { String name = "Cookie" + (++cookieCount); String value = String.valueOf(System.currentTimeMillis()); Cookie cookie = new Cookie(name, value); response.addCookie(cookie); response.getWriter().println("A cookie has been created successfully!"); } }Each time when you click the link ‘Add Cookies’, a new cookie is created and sent to the browser. Create the ReadCookiesServlet class to read all cookies visible to the application, with the following code:
package net.codejava; import java.io.IOException; import java.io.PrintWriter; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.Cookie; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; @WebServlet("/read_cookies") public class ReadCookiesServlet extends HttpServlet { private static final long serialVersionUID = 1L; public ReadCookiesServlet() { } protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { PrintWriter writer = response.getWriter(); Cookie[] cookies = request.getCookies(); if (cookies == null) { writer.println("No cookies found"); } else { writer.println("Number of cookies: " + cookies.length); for (Cookie aCookie : cookies) { String name = aCookie.getName(); String value = aCookie.getValue(); writer.println(name + " = " + value); } } } }Click link ‘Read Cookies’ would give the following output in the browser:
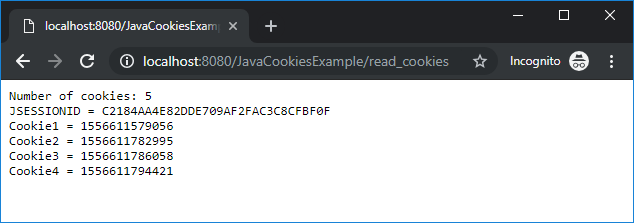
package net.codejava; import java.io.IOException; import java.io.PrintWriter; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.Cookie; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; @WebServlet("/delete_cookies") public class DeleteCookiesServlet extends HttpServlet { private static final long serialVersionUID = 1L; public DeleteCookiesServlet() { } protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { PrintWriter writer = response.getWriter(); Cookie[] cookies = request.getCookies(); if (cookies != null) { for (Cookie aCookie : cookies) { aCookie.setMaxAge(0); response.addCookie(aCookie); } writer.println("All cookies have been deleted!"); } else { writer.println("No cookies found"); } } }You can download the Java Cookies Example project in the Attachments section below.Learn more:
Using cookies to implement remember password feature
API References:
Other Java Servlet Tutorials:
- Java Servlet for beginners (XML)
- Java Servlet for beginners (Annotation)
- Java Servlet and JSP Hello World Tutorial with Eclipse, Maven and Apache Tomcat
- How to use Session in Java web application
- Java File Upload Example with Servlet
- Java File Download Servlet Example
About the Author:

Comments