How to use Session in Java web application
- Details
- Written by Nam Ha Minh
- Last Updated on 28 June 2019   |   Print Email
2. Session Management in Java
3. Getting or Creating a Session
4. Binding Data to a Session
5. Configure Session Timeout
6. Invalidate a Session
1. What is Session?
In terms of world wide web, a session indicates a period of time that a single user visits a website. A session starts when the user requests for the first page. And during a session, the user can view as many pages as he wants. The session ends if the user hasn’t requested any pages for a given amount of time (timeout). The session timeout varies, depend on server configuration – typically from 15 to 30 minutes.Because the HTTP protocol is stateless, the server can track session via cookies, URL rewriting or hidden form fields – to identify a single user across different requests. Session tracking using cookies is the primary mechanism. In case the user’s web browser doesn’t support cookies, then URL rewriting or hidden form fields can be used.In web development, programmers use session mechanism to manage user’s information that persists only in particular session, such as authentication state, username or any data that need to be shared across requests.
2. Session Management in Java
In Java, a HttpSession object represents the session of a particular user. Note that HttpSession is an interface defined in the javax.servlet package, whereas the actual implementation is injected to the HttpServletRequest by the servlet container (i.e. the server like Tomcat).You can store user-related information in a session in form of key and value pairs. The HttpSession interface defines the setAttribute(key, value) method to store a key-value entry and getAttribute(key) method to get value of a specified key.
3. Getting or Creating a Session
By default, a session is automatically created when the user visits the website. To obtain the HttpSession object representing the user’s session, invoke the getSession() method of the HttpServletRequest interface in doGet() or doPost() method of a Java Servlet. For example:protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { HttpSession session = request.getSession(); // work with the session... }Note that the HttpServletRequest .getSession() method returns the current session associated with the request, or create a new one if the request doesn’t have a session. That means the returned HttpSession object is always not null.To get a session without creating a new one if not exist, you can use invoke getSession(false) on the HttpServletRequest:
HttpSession session = request.getSession(false); if (session != null) { // a session exists } else { // no session }In this case, the returned value can be null if no session exists – hence the if-else check for nullability is needed. That also means getSession() is equivalent to getSession(true).For your reference, the following Java Servlet prints the session ID, creation time and last accessed time of the current session:
package net.codejava; import java.io.*; import java.util.*; import javax.servlet.*; import javax.servlet.http.*; @WebServlet("/test_session") public class TestSessionServlet extends HttpServlet { private static final long serialVersionUID = 1L; public TestSessionServlet() { super(); } protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { HttpSession session = request.getSession(); PrintWriter writer = response.getWriter(); writer.println("Session ID: " + session.getId()); writer.println("Creation Time: " + new Date(session.getCreationTime())); writer. println("Last Accessed Time: " + new Date(session.getLastAccessedTime())); } }You can run this servlet for testing purpose. The result would look like this:
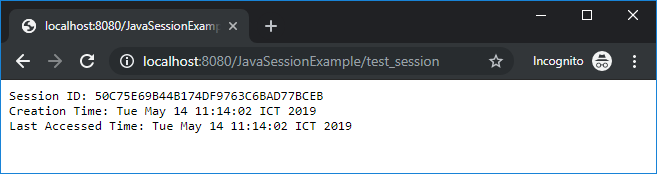
4. Binding Data to a Session
Store value in session:To store a value in a session, use the method setAttribute(key, value) of the HttpSession object. For example, the following statement stores username of the user:session.setAttribute("username", "Daniel Tran");Here, the key is username and value is Daniel Tran. Data stored in a session is managed by the server and will be deleted when the session ends.You can store any kind of object in the session. For example, the following code stores a List of Student objects in the session:
List<Student> students = studentDao.getStudents(); session.setAttribute("listStudent", students);NOTES:Each user is associated with different HttpSession object, so the values stored for user #1 are different than the values stored for user #2 - although the key is the same.If the key is already associated with a value, then the old value is replaced by the new one. So you can use the setAttribute() method to update value in the session.
Read value from session in Java Servlet:
To get value from a session, use the getAttribute(key) method of the HttpSession object. For example, the following code gets value of the username attribute from the session:String username = (String) session.getAttribute("username");We need a cast to String type because the getAttribute() method always returns a value of Object type.The following statement reads a List collection from the session:
List<Student> listStudents = (List<Student>) session.getAttribute("listStudent");Note that the getAttribute(key) method will return null value if the given key is not found in the session.
Read value from session in JSP:
In JSP, to read and display value of an attribute stored in the session, just use EL (expression language) as follows:<%@ page language="java" %> <html> <body> <p>Username: ${username}</p> </body> </html>Here, the JSP processor will find an attribute username in possible scopes, including the session. Or you can specify the session scope explicitly in the expression:
<p>Username: ${sessionScope['username']}</p>
Remove value from session:
To delete a value associated with a key from the session, use the removeAttribute(key) method. For example:session.removeAttribute("username");This statement removes the value associated with the attribute username.
5. Configure Session Timeout
If a user has been idle (has not made any requests) for a given amount of time, his session expires – which means all the data bound to his session is removed from the server – the session is destroyed. Each server has different default value for global session timeout, e.g. 30 minutes in Apache Tomcat.You can set session timeout for an individual web application by modifying its web deployment descriptor file (web.xml). For example:<?xml version="1.0" encoding="UTF-8"?> <web-app...> <session-config> <session-timeout>15</session-timeout> </session-config> </web-app>This overrides the global session timeout set by the server, and sets session timeout to 15 minutes.You can set timeout value for an individual session programmatically like this:
session.setMaxInactiveInterval(300);This sets the timeout for the current session, for 300 seconds.Read this article for more details about setting session timeout in Java.
6. Invalidate a Session
By default, a session is destroyed only after the user has been idle for a timeout period. In case you want to destroy an individual session immediately, call the invalidate() method like this:session.invalidate();This removes any objects bound to the session and destroy it. API Reference:
Related Java Session Tutorials:
- How to set Session Timeout for Java web application
- How to configure session timeout in Tomcat
- How to code login and logout with Java Servlet, JSP and MySQL
- How to Code Hit Counter for Java web application
Other Java Servlet Tutorials:
- Java Servlet for beginners (XML)
- Java Servlet for beginners (Annotation)
- Java Servlet and JSP Hello World Tutorial with Eclipse, Maven and Apache Tomcat
- How to use Cookies in Java web application
- Java File Upload Example with Servlet
- Java File Download Servlet Example
About the Author:

Comments