JList basic tutorial and examples
- Details
- Written by Nam Ha Minh
- Last Updated on 06 July 2019   |   Print Email
JList is a Swing component with which we can display a list of elements. This component also allows the user to select one or more elements visually. This article shows how to work with JList and proceeds to show some examples.
We will write code to achieve a JList output like:
Output
Table of Contents:
5. Developing a Selection Listener
1. Developing A Simple JList:
Let us now build a GUI with a JList. Let us say we want to show a list of countries. As with other Swing components, the data for a JList is held in a model. This is represented by ListModel interface in the Swing API. The API provides a default implementation of this class named DefaultListModel. More often than not, we would want to display a list of homogeneous elements.
Using the DefaultListModel:
Let us now see how to create and use the DefaultListModel.
Note that, since version 1.7, the API for JList allows the ability to create a JList with a parameterized type. So, we will use that syntax to create a JList that will accept a List<String>:
DefaultListModel<String> listModel = new DefaultListModel<>(); listModel.addElement("USA"); listModel.addElement("India"); listModel.addElement("Vietnam"); listModel.addElement("Canada"); listModel.addElement("Denmark"); listModel.addElement("France"); listModel.addElement("Great Britain"); listModel.addElement("Japan");
The preceding piece of code is quite simple. We create an instance of the DefaultListModel class by declaring it as accepting only String values using the parameterized syntax.
Then, we can use this model to create a JList:
JList<String> countryList = new JList<>(listModel);
It is important to note that the JList declaration and usage should also be parameterized.
Running the Sample:
Let us provide the full source code and run it:
package net.codejava.swing; import javax.swing.DefaultListModel; import javax.swing.JFrame; import javax.swing.JList; import javax.swing.SwingUtilities; public class JListExample extends JFrame { private JList<String> countryList; public JListExample() { //create the model and add elements DefaultListModel<String> listModel = new DefaultListModel<>(); listModel.addElement("USA"); listModel.addElement("India"); listModel.addElement("Vietnam"); listModel.addElement("Canada"); listModel.addElement("Denmark"); listModel.addElement("France"); listModel.addElement("Great Britain"); listModel.addElement("Japan"); //create the list countryList = new JList<>(listModel); add(countryList); this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); this.setTitle("JList Example"); this.setSize(200,200); this.setLocationRelativeTo(null); this.setVisible(true); } public static void main(String[] args) { SwingUtilities.invokeLater(new Runnable() { @Override public void run() { new JListExample(); } }); } }
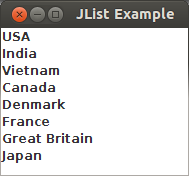
Initial Output
2. Adding a Scrollpane:
Let us now try and resize the frame. We will get the following output:
Resized Frame without Scrollbar
But, where are the rest of the countries? They have disappeared from the display. But, ideally, we would want the scrollbar to appear in this case. To make this happen, we have to add the list to a scrollpane instead of adding it directly:
add(new JScrollPane(countryList));
Let us now run the program:
Output with Scrollbar
3. Set Selection Mode for JList
The selection mode defines the way elements can be selected. There are totally 3 selection modes available to be set for JList:
SINGLE_SELECTION:
This mode specifies that only a single item can be selected at any point of time.
SINGLE_INTERVAL_SELECTION:
This mode specifies that multiple items can be selected, but they have to be contiguous. Items can be selected contiguously by pressing down the shift key and selecting elements with the mouse.
MULTIPLE_INTERVAL_SELECTION:
This mode is the default mode. This mode specifies that multiple items can be selected and they may or may not be contiguous.
Let us now run the program and select multiple items. We will hold down the shift key and click with mouse to select contiguous items. For non-contiguous items, we need to press down the ctrl key and select with the mouse.
We get the following output:
Output with Multiple Items Selected
Changing the Selection Mode:
Let us now try and change the selection mode. This can be done as follows:
countryList.setSelectionMode(ListSelectionModel.SINGLE_SELECTION);
We make a call to the setSelectionMode() method and make use of the constants declared in the ListSelectionModel to set our selection mode. Let us now run the program with this selection mode and try to select multiple items:
Single Selection Mode
When we run the program with this change and try to select multiple items (by pressing down either the shift or ctrl key), we find out that we are unable to select multiple items.
4. Event Handlers:
Let us now try and develop event handlers for JList. Event handling in JList is very similar to that of other Swing components. We register an event listener and are notified when the event happens.
5. Developing A Selection Listener for JList
Let us write a selection listener for JList. Knowing the currently selected item(s) will be one of the most useful events to know. Note that, the user might have selected one or more item(s) in the list. Also, the user may deselect a selected item. If the user had earlier selected only one item and now deselected the same, there would be no selection left in the list.
The selection listener for the list fires the event even in the case of user deselecting an item. This might be quite useful in certain scenarios.
Let us now add the following listener code:
countryList.addListSelectionListener(new ListSelectionListener() { @Override public void valueChanged(ListSelectionEvent e) { if(!e.getValueIsAdjusting()) { final List<String> selectedValuesList = countryList.getSelectedValuesList(); System.out.println(selectedValuesList); } } });
The above code registers a ListSelectionListener using the addListSelectionListener() method. We use an anonymous inner class to implement the event listener interface. We implement the valueChanged() method. We call the handy method getSelectedValuesList() on the JList instance which returns a List<String>, as in our case, we had declared the JList to contain only String values. For illustration purposes, we simply print this list on the console which prints the list of values selected.
Now, run the program and select a few items (single, multiple, contiguous, non-contiguous) and check the output on the console. Now, deselect all items in the list and check the output on the console. It will print an empty list.
We use extra if condition in the code which checks if the list is still adjusting the status. If it is not, we retrieve the selected items.
Here is the full source code of our GUI:
package net.codejava.swing; import java.util.List; import javax.swing.DefaultListModel; import javax.swing.JFrame; import javax.swing.JList; import javax.swing.JScrollPane; import javax.swing.SwingUtilities; import javax.swing.event.ListSelectionEvent; import javax.swing.event.ListSelectionListener; /** * JList basic tutorial and example * * @author wwww.codejava.net */ public class JListExample extends JFrame { private JList<String> countryList; public JListExample() { //create the model and add elements DefaultListModel<String> listModel = new DefaultListModel<>(); listModel.addElement("USA"); listModel.addElement("India"); listModel.addElement("Vietnam"); listModel.addElement("Canada"); listModel.addElement("Denmark"); listModel.addElement("France"); listModel.addElement("Great Britain"); listModel.addElement("Japan"); //create the list countryList = new JList<>(listModel); countryList.addListSelectionListener(new ListSelectionListener() { @Override public void valueChanged(ListSelectionEvent e) { if (!e.getValueIsAdjusting()) { final List<String> selectedValuesList = countryList.getSelectedValuesList(); System.out.println(selectedValuesList); } } }); add(new JScrollPane(countryList)); this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); this.setTitle("JList Example"); this.setSize(200, 200); this.setLocationRelativeTo(null); this.setVisible(true); } public static void main(String[] args) { SwingUtilities.invokeLater(new Runnable() { @Override public void run() { new JListExample(); } }); } }
Other Java Swing Tutorials:
- Java Swing Hello World Tutorial for Beginners Using Text Editor
- JFrame basic tutorial and examples
- JPanel basic tutorial and examples
- JLabel basic tutorial and examples
- JTextField basic tutorial and examples
- JButton basic tutorial and examples
- JComboBox basic tutorial and examples
- JCheckBox basic tutorial and examples
- JTree basic tutorial and examples
About the Author:

Comments
Then you need to use a custom List renderer. See example here: www.codejava.net/.../jlist-custom-renderer-example