AWS Java SDK S3 Create Folder Examples
- Details
- Written by Nam Ha Minh
- Last Updated on 03 January 2022   |   Print Email
In this Java Amazon S3 tutorial, I’d like to share some code examples for programmatically creating folders in a bucket on Amazon S3 server, using AWS SDK for Java.
To follow this guide, you must have an AWS SDK for S3 set up for your Java Maven project. If not, kindly follow this article.
1. What is actually a Folder in Amazon S3?
In Amazon S3, everything inside a bucket is object. That means there’s no folder like folder which you see in the normal file system. In S3, a “folder” is actually an empty object with delimiter “/” at the end of the objet key, for examples:programming/
programming/java/ programming/python/Though you can see folders on AWS Management console as shown below, they are actually objects with type “folder” - not folders like in hierarchical file system: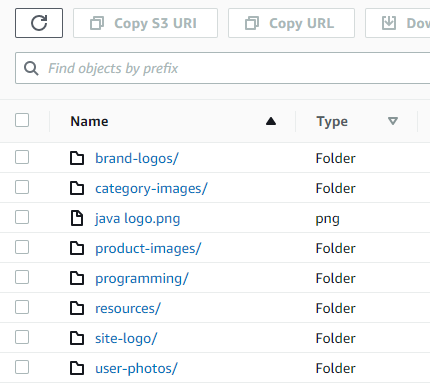
2. Create a Folder in a S3 Bucket
The following example program shows the code that uses AWS SDK S3 to create a folder named projects/docs/ inside the bucket code-java-bucket:package net.codejava.aws; import software.amazon.awssdk.core.sync.RequestBody; import software.amazon.awssdk.services.s3.S3Client; import software.amazon.awssdk.services.s3.model.PutObjectRequest; public class CreateFolderExample { public static void main(String[] args) { String bucketName = "code-java-bucket"; String folderName = "projects/docs/"; S3Client client = S3Client.builder().build(); PutObjectRequest request = PutObjectRequest.builder() .bucket(bucketName).key(folderName).build(); client.putObject(request, RequestBody.empty()); System.out.println("Folder " + folderName + " is ready."); } }You see, the code is self-explanatory. It sends a PutObjectRequest to S3 server for creating an empty object. You can specify any hierarchy-like folder name, as it’s actually object key.
3. Wait Until the Folder Exists
package net.codejava.aws; import software.amazon.awssdk.core.sync.RequestBody; import software.amazon.awssdk.core.waiters.WaiterResponse; import software.amazon.awssdk.services.s3.S3Client; import software.amazon.awssdk.services.s3.model.HeadObjectRequest; import software.amazon.awssdk.services.s3.model.HeadObjectResponse; import software.amazon.awssdk.services.s3.model.PutObjectRequest; import software.amazon.awssdk.services.s3.waiters.S3Waiter; public class CreateFolderExample { public static void main(String[] args) { String bucketName = "nam-public-images"; String folderName = "asia/vietnam/"; S3Client client = S3Client.builder().build(); PutObjectRequest request = PutObjectRequest.builder() .bucket(bucketName).key(folderName).build(); client.putObject(request, RequestBody.empty()); S3Waiter waiter = client.waiter(); HeadObjectRequest requestWait = HeadObjectRequest.builder() .bucket(bucketName).key(folderName).build(); WaiterResponse<HeadObjectResponse> waiterResponse = waiter.waitUntilObjectExists(requestWait); waiterResponse.matched().response().ifPresent(System.out::println); System.out.println("Folder " + folderName + " is ready."); } }This code will wait until the folder exists, so you can perform your custom logics afterward.That’s a couple of examples about creating folders on Amazon S3. To see the coding in action, kindly watch my video on YouTube:
Related AWS Java SDK Tutorials:
- How to Generate AWS Access Key ID and Secret Access Key
- How to setup AWS SDK for Java for Amazon S3 Development
- AWS Java SDK S3 List Buckets Example
- AWS Java SDK S3 List Objects Examples
- AWS Java SDK S3 Create Bucket Examples
- Upload File to S3 using AWS Jav SDK - Java Console Program
- Upload File to S3 using AWS Java SDK - Java Servlet JSP Web App
- Spring Boot File Upload to Amazon S3 Example
- AWS Java SDK Download File from S3 Example
- AWS Java SDK S3 Delete Objects Examples
- AWS Java SDK S3 Delete Buckets Examples
About the Author:
