JSTL Core Tag c:forTokens Example
- Details
- Written by Nam Ha Minh
- Last Updated on 31 August 2019   |   Print Email
JSTL <c:forTokens> Syntax:
<c:forTokens
items="<string>"
delims="<string>"
begin="<int>"
end="<int>"
step="<int>"
var="<string>"
varStatus="<string>">
...
</c:forTokens>
Name | Required | Type | Description |
items | True | java.lang.String | String of the tokens to iterate. This string should have a delimiter. |
delims | True | java.lang.String | Delimiters which separate the tokens. |
begin | False | int | Iteration begins at the index specified in this attribute. |
end | False | int | Iteration ends at the index specified in this attribute. |
step | False | int | Step value for the iteration specified in this attribute. |
var | False | java.lang.String | Name of the variable which holds the current item in the iteration and has nested visibility. |
varStatus | False | java.lang.String | Name of the scoped variable which holds the loop status of the current iteration. This variable is of type javax.servlet.jsp.jstl.core.LoopTagStatusand has nested visibility. |
JSTL <c:forTokens> Example:
The below is a fully working example to demonstrate the usage of <c:forTokens> tag:<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%> <%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1"> <title><c:forTokens> Demo</title> </head> <body> <h1><c:forTokens> Demo</h1> <form name="forTokensForm" action="${pageContext.request.contextPath}/tag-types/core/for-tokens.jsp" method="POST"> Enter some text with delimiter: <input type="text" name="delimText" /><br/> Enter the delimiter: <input type="text" name="delim" size="3"/><br/> <input type="submit" value="Tokenize"/> </form> <br/><br/> Your tokens: <br/> <c:forTokens items="${param.delimText}" delims="${param.delim}" var="myToken"> <c:out value="${myToken}"/><br/> </c:forTokens> </body> </html>The above example takes a string from the user along with the delimiter which separates the string and does tokenization using the delimiter user supplied.The following example iterates over a string of delimited values. The delimiter used is comma (,).
<c:forTokens items="One,Two,Three,Four" delims="," var="myCount"> <c:out value="${myCount}"/><br/> </c:forTokens>
Note that in the above example we only used one delimiter to print the value of the tokens. However, we can use multiple delimiters to print the values of the tokens separated by different delimiter characters. The following example shows this:
<c:forTokens items="One,Two,Three,Four.Five.Six" delims=",." var="myCount"> <c:out value="${myCount}"/><br/> </c:forTokens>
In the above example we have two delimiters (i.e., comma and a period). This example prints all the token values.
In case if you omit the period as shown in the below example, it will only print One Two Three as tokens and consider Four Five Six as single token since we have only specified comma as a delimiter.
<c:forTokens items="One,Two,Three,Four.Five.Six" delims="," var="myCount"> <c:out value="${myCount}"/><br/> </c:forTokens>
The attributes begin, end, step, var andvarStatus behave similar to the respective counterparts of the <c:forEach> tag. However, in this case they are applicable to the tokens.
Output:
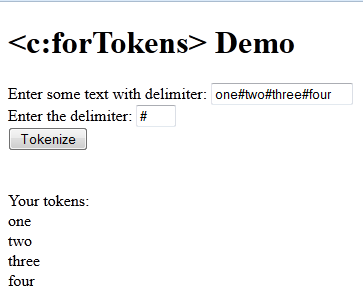
Recommended Usage of JSTL <c:forTokens> tag:
<c:forTokens> is useful in scenarios where a string needs to be separated into tokens using one or more delimiters. Particularly handy when we have more than one delimiter in the string we need to tokenize in which case we can specify more than one delimiter.
Other JSTL Core Tags:
if | catch | choose | forEach | import | out | param | redirect | remove | set | urlAbout the Author:

Comments