JSTL Format Tag fmt:formatNumber Example
- Details
- Written by Nam Ha Minh
- Last Updated on 31 August 2019   |   Print Email
JSTL <fmt:formatNumber> Syntax:
<fmt:formatNumber
value="<string>"
type="<string>"
pattern="<string>"
currencyCode="<string>"
currencySymbol="<string>"
groupingUsed="<string>"
maxIntegerDigits="<string>"
minIntegerDigits="<string>"
maxFractionDigits="<string>"
minFractionDigits="<string>"
var="<string>"
scope="<string>"/>
Attributes:
Name | Required | Type | Description |
value | False | java.lang.String | Numeric value to be formatted. |
type | False | java.lang.String | Determines whether the value is formatted as a number, currency or percentage. |
pattern | False | java.lang.String | Formatting pattern. |
currencyCode | False | java.lang.String | The currency code to be used if the type attribute is currency. |
currencySymbol | False | java.lang.String | The currency symbol to be used if the typeattribute is currency. |
groupingUsed | False | java.lang.String | Whether any grouping separated to be used when formatting the output. |
maxIntegerDigits | False | java.lang.String | Maximum number of digits in the integer portion. |
minIntegerDigits | False | java.lang.String | Minimum number of digits in the integer portion. |
maxFractionDigits | False | java.lang.String | Maximum number of digits in the fraction portion. |
minFractionDigits | False | java.lang.String | Minimum number of digits in the fraction portion. |
var | False | java.lang.String | Name of the variable which stores the formatted result. |
scope | False | java.lang.String | Scope to store the var. |
JSTL <fmt:formatNumber> Example:
The below JSP code takes account balances and displays it using various formats.<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <%@ taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1"> <title><fmt:formatNumber> Demo</title> </head> <body> <h1><fmt:formatNumber> Demo</h1> The Account Balance can be displayed using various ways: <c:set var="accountBalance" value="9500.60" /> <p>as it is: <strong><fmt:formatNumber value="${accountBalance}" type="currency"/></strong></p> <p>max. integer digits 3: <strong> <fmt:formatNumber type="number" maxIntegerDigits="3" value="${accountBalance}" /> </strong></p> <p>max. fraction digits 1: <strong> <fmt:formatNumber type="number" maxFractionDigits="3" value="${accountBalance}" /> </strong></p> <p>no grouping: <strong> <fmt:formatNumber type="number" groupingUsed="false" value="${accountBalance}" /> </strong></p> <p>percent with max. integer digits 3: <strong> <fmt:formatNumber type="percent" maxIntegerDigits="3" value="${accountBalance}" /> </strong></p> <p>percent with min. fraction digits 10: <strong> <fmt:formatNumber type="percent" minFractionDigits="10" value="${accountBalance}" /> </strong></p> <p>pattern ###.###E0: <strong> <fmt:formatNumber type="number" pattern="###.###E0" value="${accountBalance}" /> </strong></p> <p>Account Balance in USA : <fmt:setLocale value="en_US"/> <strong><fmt:formatNumber value="${accountBalance}" type="currency"/></p></strong> <p>Account Balance in Canada : <fmt:setLocale value="fr_CA"/> <strong><fmt:formatNumber value="${accountBalance}" type="currency"/></p></strong> </body> </html>
Output:
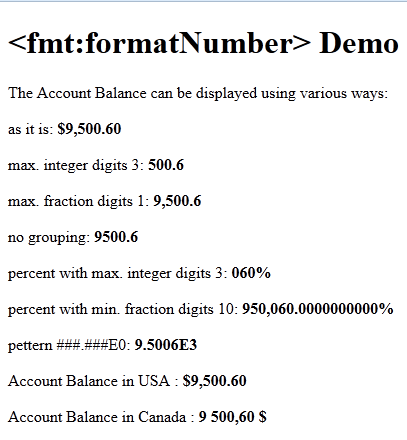
Recommended Usage of JSTL <fmt:formatNumber> tag:
Mostly used to format numbers, currency and percentage values. Attributes are provided to control the number of digits to be displayed in the decimal and fractional parts. We can enable or disable grouping which is used to display commas (,) to separate thousand groups. We can specify various patterns to display the values. The following table summarizes the representations to be used in the pattern.
Symbol | Description |
0 | Represents digits. |
E | Represents exponential. |
# | Represents a single digit. |
. | Place holder for the decimal separator. |
, | Place holder for grouping separator. |
; | Separates formats. |
- | Default negative prefix. |
% | Multiplies by 100 and displays as percentage. |
? | Multiples by 1000 and displays as milli. |
X | Indicates that any other character can be used in the prefix or suffix |
' | Used to quote special characters in the prefix or suffix. |
| Represents currency symbol. Replaced by currency symbol. |
Other JSTL Format Tags:
bundle | formatDate | message | param | parseDate | parseNumber | requestEncoding | setBundle | setLocale | setTimeZone | timeZone
About the Author:

Comments