How to create File picker component in Java Swing
- Details
- Written by Nam Ha Minh
- Last Updated on 04 July 2019   |   Print Email

1. Source code of JFilePicker component
There are two source file that forms the file picker component:- JFilePicker: the file picker component class.
- FileTypeFilter: represents a file extension filter added to the JFileChooser class (See the article: Add file filter for JFileChooser dialog).
package net.codejava.swing; import java.awt.FlowLayout; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import javax.swing.JButton; import javax.swing.JFileChooser; import javax.swing.JLabel; import javax.swing.JPanel; import javax.swing.JTextField; public class JFilePicker extends JPanel { private String textFieldLabel; private String buttonLabel; private JLabel label; private JTextField textField; private JButton button; private JFileChooser fileChooser; private int mode; public static final int MODE_OPEN = 1; public static final int MODE_SAVE = 2; public JFilePicker(String textFieldLabel, String buttonLabel) { this.textFieldLabel = textFieldLabel; this.buttonLabel = buttonLabel; fileChooser = new JFileChooser(); setLayout(new FlowLayout(FlowLayout.CENTER, 5, 5)); // creates the GUI label = new JLabel(textFieldLabel); textField = new JTextField(30); button = new JButton(buttonLabel); button.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent evt) { buttonActionPerformed(evt); } }); add(label); add(textField); add(button); } private void buttonActionPerformed(ActionEvent evt) { if (mode == MODE_OPEN) { if (fileChooser.showOpenDialog(this) == JFileChooser.APPROVE_OPTION) { textField.setText(fileChooser.getSelectedFile().getAbsolutePath()); } } else if (mode == MODE_SAVE) { if (fileChooser.showSaveDialog(this) == JFileChooser.APPROVE_OPTION) { textField.setText(fileChooser.getSelectedFile().getAbsolutePath()); } } } public void addFileTypeFilter(String extension, String description) { FileTypeFilter filter = new FileTypeFilter(extension, description); fileChooser.addChoosableFileFilter(filter); } public void setMode(int mode) { this.mode = mode; } public String getSelectedFilePath() { return textField.getText(); } public JFileChooser getFileChooser() { return this.fileChooser; } }Source code of FileTypeFilter class:
package net.codejava.swing; import java.io.File; import javax.swing.filechooser.FileFilter; public class FileTypeFilter extends FileFilter { private String extension; private String description; public FileTypeFilter(String extension, String description) { this.extension = extension; this.description = description; } @Override public boolean accept(File file) { if (file.isDirectory()) { return true; } return file.getName().toLowerCase().endsWith(extension); } public String getDescription() { return description + String.format(" (*%s)", extension); } }
2. How to use JFilePicker class
The constructor of JFilePicker class accepts two parameters:
- textFieldLabel: label for the text field.
- buttonLabel: label for the button.
JFilePicker filePicker = new JFilePicker("Pick a file", "Browse...");
Next, we specify mode of the dialog, open or save:
filePicker.setMode(JFilePicker.MODE_OPEN);
or:
filePicker.setMode(JfilePicker.MODE_SAVE);File extension filters can be added likes this:
filePicker.addFileTypeFilter(".jpg", "JPEG Images"); filePicker.addFileTypeFilter(".mp4", "MPEG-4 Videos");And the selected file’s path can be retrieved using getSelectedFilePath()method:
String filePath = filePicker.getSelectedFilePath();The JFilePicker class also provides a mean to access the JFileChooser class directly, in case you want to do more tweaks:
JFileChooser fileChooser = filePicker.getFileChooser(); fileChooser.setCurrentDirectory(new File("D:/"));
3. JFilePicker component example program
And following is an example program that uses the JFilePicker component we created above. It is a very simple JFrame which contains only the file picker component. Here is the source code:package net.codejava.swing; import java.awt.FlowLayout; import java.io.File; import javax.swing.JFileChooser; import javax.swing.JFrame; import javax.swing.SwingUtilities; public class TestJFilePicker extends JFrame { public TestJFilePicker() { super("Test using JFilePicker"); setLayout(new FlowLayout()); // set up a file picker component JFilePicker filePicker = new JFilePicker("Pick a file", "Browse..."); filePicker.setMode(JFilePicker.MODE_SAVE); filePicker.addFileTypeFilter(".jpg", "JPEG Images"); filePicker.addFileTypeFilter(".mp4", "MPEG-4 Videos"); // access JFileChooser class directly JFileChooser fileChooser = filePicker.getFileChooser(); fileChooser.setCurrentDirectory(new File("D:/")); // add the component to the frame add(filePicker); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setSize(520, 100); setLocationRelativeTo(null); // center on screen } public static void main(String[] args) { SwingUtilities.invokeLater(new Runnable() { @Override public void run() { new TestJFilePicker().setVisible(true); } }); } }When running, the program looks like the following screenshot:

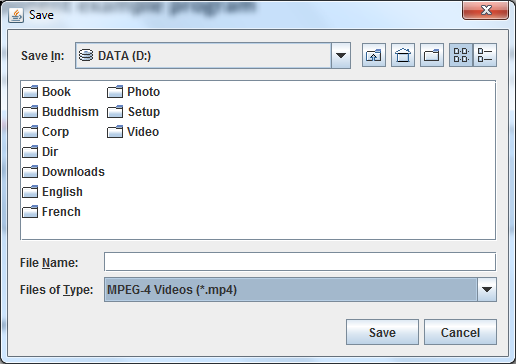

Related Swing File Chooser Tutorials:
- How to add file filter for JFileChooser dialog
- Show save file dialog using JFileChooser
- Show simple open file dialog using JFileChooser
Other Java Swing Tutorials:
- Java Swing Hello World Tutorial for Beginners Using Text Editor
- JFrame basic tutorial and examples
- JPanel basic tutorial and examples
- JLabel basic tutorial and examples
- JTextField basic tutorial and examples
- JButton basic tutorial and examples
About the Author:

Comments
thanks for sharing in advance. thank you.
You can disable all files filter easily, just calling:
setAcceptAllFileFilterUsed(false)