Spring Boot automatic restart using Spring Boot DevTools
- Details
- Written by Nam Ha Minh
- Last Updated on 26 February 2020   |   Print Email
1. What is Spring Boot automatic restart?
When you make changes to Java code, Spring Boot DevTools will restart the embedded server to reflect the changes. It is full, automatic restart but quick enough – much faster than “cold” restart – because the things do not change (e.g. jars) are already loaded in a separate classloader.Note that automatic restart is different than automatic reload (or live reload) which reloads only changes for static content (e.g. Thymeleaf templates) in the browser. And this post focuses on Spring Boot automatic restart.2. Enable Automatic Restart for Spring Boot project
To use Spring Boot DevTools, you must declare the following dependency into your project’s pom.xml file:<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <optional>true</optional> </dependency>This dependency is specified as optional so the Spring DevTools will not be used in projects/modules that depend on yours. If you have only one project, you don’t have to use the optional attribute.If you use Spring Tool Suite IDE, enable Spring Boot DevTools is easy: right-click on the project, then click Spring > Add DevTools from the context menu:
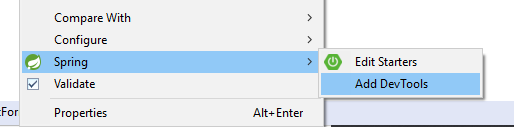
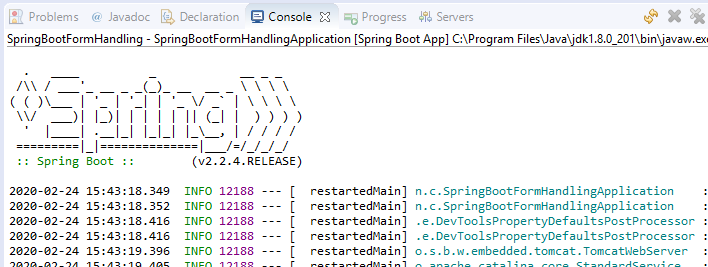
3. What changes will trigger automatic restart?
By default, any changes (create, update or delete) you made to any files in the project’s classpath (typically files under src/main directory) will trigger Spring DevTools to restart the application to update the changes. Actually, Spring Boot DevTools monitors changes for all entries under the project’s classpath.If you want to include additional paths (files that are not on the classpath) to be monitored for changes, specify the following property in the application.properties file:spring.devtools.restart.additional-paths=g:/Java/WebServices,data
The example above, this will add two directories outside the project’s classpath to be monitored for changes by Spring DevTools. The first path points to an absolute path on the computer, and the second one points to the data directory under the project’s root.Note that changes to tests code (files under src/test/java) will not trigger automatic restart because testing code should be executed on request - not auto.4. Exclude paths from automatic restart
By default, changes to resources in the following paths won’t trigger an automatic restart: /META-INF/maven, /META-INF/resources, /resources, /static, /public, and /templates.If you want to override this exclusion, specify the spring.devtools.restart.exclude property in the application.properties file. For example:spring.devtools.restart.exclude=static/**
Then changes to any files under src/main/resources/static will not trigger automatic restart.In case you want to keep the default exclusion paths and add additional paths for exclusion, use the spring.devtools.restart.additional-exclude property instead.5. Remove Spring Boot DevTools
By default, the jar files of Spring Boot DevTools are not included in your project’s package (JAR/WAR). If you no longer want to use automatic restart, just remove the spring-boot-devtools dependency in the pom.xml file. In case you use Spring Tool Suite, a quick way is right-click on the project, then click Spring > Remove DevTools: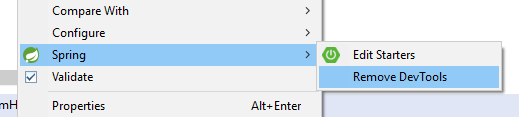
Other Spring Boot Tutorials:
- Spring Boot Hello World Example
- Spring Boot Form Handling Tutorial with Spring Form Tags and JSP
- Spring Boot Hello World RESTful Web Services Tutorial
- How to create a Spring Boot Web Application (Spring MVC with JSP/ThymeLeaf)
- Spring Boot - Spring Data JPA - MySQL Example
- Spring Boot CRUD Example with Spring MVC – Spring Data JPA – ThymeLeaf - Hibernate - MySQL
- How to use JDBC with Spring Boot
- Spring Boot CRUD Web Application with JDBC - Thymeleaf - Oracle
- Spring Boot RESTful CRUD API Examples with MySQL database
- How to package Spring Boot application to JAR and WAR
About the Author:

Comments